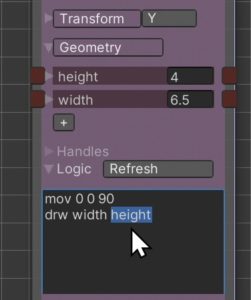
Turtle Script creates custom 2D shapes
- Scripts are parsed within the Logic of 2D shape Geometry.
- Use a new line for each turtle command.
- Node parameters and math functions can replace script values
- The turtle can only read/set parameters on its own node.
Turtle commands fall into a few general categories
- explicit coordinates: mov, drw, dir, bezier…
- relative coordinates: rmov, rdrw, arc…
- self-steering: fwd, back, left, right, turn…
- logic: let, set, loop, if…
EXPLICIT COORDINATESthe following commands use 2D coordinates and a universal direction, |
|
mov x y (a) | move to x y (degrees) teleport turtle to location (face direction) |
drw x y | draw to location x y |
dir a | direction angle in degreesdir 0 is east, dir 90 is north |
bezier ax ay ahx ahy bhx bhy bx by segments | cubic bezier with 2 handles startXY handle1 handle2 endXY segments bezier 0 0 0 1 1 1 1 0 16 |
molding type ax ay bx by segments tension | point-to-point tension curves type* start end segments tension tension is normalized 0-1 *types: cove, ovolo, cymarecta, cymareversa, dome molding ovolo 0 1 1 0 16 .5 |
RELATIVE COORDINATESthe following commands use 2D coordinates relative to the turtle’s current position and orientation |
|
newp | new point creates a vertex in-situ restarts the UV map |
rmov dx dy | relative move offset-x offset-y teleport to offset restarts the UV map *shape must be OPEN |
rdrw dx dy | relative draw offset-x offset-y |
arcl angle radius segments | draw an arc forward and to the left angle of the full arc, in degrees radius of the arc, centered to the left segments (int) arcl 90 1 8 |
arcr angle radius segments | draw an arc forward and to the right angle of the full arc, in degrees radius of the arc, centered to the right segments (int) arcr 90 1 8 |
ROTATION AND STEERINGthe following commands ‘steer’ the turtle according to it’s current rotation and position |
|
movfwd c (d) | move forward value (offset) teleport in the facing direction *shape must be OPEN |
fwd c (d) | forward value (offset) |
back c (d) | back value (offset) |
right c (d) | right value (offset) |
left c (d) | left value (offset) |
turnl a | rotate counter-clockwise degrees |
turnr a | rotate clockwise degrees |
LOGICthe following commands add mathf, temp variables, loops, and conditional logic |
|
closed closed true closed false |
adds a segment connecting the last point to the first removes overlapped/enclosed vertices *required for ‘manifold topology’ *changes options under Shape Output *also a bool switch under Shape Output |
let | create a temporary variable (float) let var value let myTemp 80 |
set | set value on a parameter or variable set parameter value set var value set myParam 16 |
loop repeat (count) (step) | open a loop and repeat (int) until done (count) create temp var (int) = the loop value (step) steps through the count by (int) loop 360 rotate 90 ... |
endloop | terminate loop |
if conditional | open a conditional directive when trueif parameter GT value endif |
var GT value var LT value var GE value var LE value var EQ value var NE value |
greater than less than greater or equal lesser or equal equal not equal |
if bool | run conditional when bool is true |
if !bool | run conditional when bool is false |
endif | terminate conditional |
// | comment line |
+ – * / (…) |
add subtract (negative) multiply divide order of operations |
mathf functions | function returns a valuelet hypotenuse sqrt(pow(width,2)+pow(height,2)) |
parameter | the value of a parametermov width 0 |
DetailLevel | read-only parameter from graph: 0-1 *reduces segments on all nodes: 0.5 is 50% *generates LOD models by reducing vertices if DetailLevel LT .67 |
tips
Each line the turtle draws, creates a new edge and vertex.
When 2D shapes combine into a 3D mesh, the turtle defines how the texture will wrap the model. On the generated mesh U follows the Plan shape and direction, V follows the Section.
‘Steering’ the turtle (ie: fwd, turnl/r) creates clean UV wrapping – easiest method to follow details on a texture map, or create specific vertices on a mesh without stretching the texture.
Unity removes unnecessary vertices to optimize the mesh – which is bad if the mesh needs to deform around a plan. A row of tiny offset points can force vertices in an apparently straight line segment.
Example scripts
The best way to learn AX Turtle Script is to open the Logic panel on any 2D shape to see how it was made.
![]() | mov width/2 0 90 drw 0 height/2 drw –width/2 0 drw 0 –height/2 closed true |
![]() | if shift_origin molding cove –width height 0 0 segs tension endif if !shift_origin molding cove 0 height width 0 segs tension endif closed false |
![]() | if inputHypotenuse if solveWidth set width Sqrt(hypotenuse*hypotenuse–height*height) endif set height Sqrt(hypotenuse*hypotenuse–width*width) endif set hypotenuse Sqrt(width*width+height*height) set theta Atan2(height,width) mov 0 0 90 right width fwd height closed true |
![]() | if bX GE aX let mdX abs(bX–aX)/(segs-1) endif if bX LT aX let mdX -abs(bX–aX)/(segs-1) endif if bY GE aY let mdY abs(bY–aY)/(segs-1) endif if bY LT aY let mdY -abs(bY–aY)/(segs-1) endif if cX GE bX let ndX abs(cX–bX)/(segs-1) endif if cX LT bX let ndX -abs(cX–bX)/(segs-1) endif if cY GE bY let ndY abs(cY–bY)/(segs-1) endif if cY LT bY let ndY -abs(cY–bY)/(segs-1) endif mov aX aY loop segs i mov aX+mdX*i aY+mdY*i drw bX+ndX*i bY+ndY*i endloop Output Shape: open |